This topic includes these sections:
Overview
INtime Connector Device (or XCNT) is a virtual device driver for INtime Network7 TCP/IP stack. Another application can connect to the device to send and receive Ethernet frames to and from the INtime network stack.
The most common use of XCNT is to share a single NIC device with Network7 stack and HPE application (figure below), but XCNT can be used for other applications.
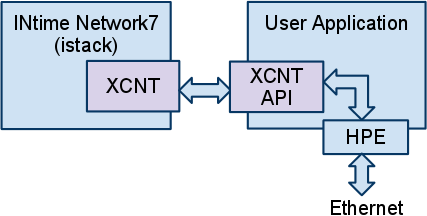
The Connector Device in the INtime Network
The connector device will appear as “xcntn” in the INtime Network where n is the instance number. For example, the first connector device will be “xcnt0”.
Connector API Calls
The following calls are implemented in the XCNT API, which is called from the user application to send and receive packets from the network stack.
xcntOpenConnector |
Establish a connection to the Connector Device |
xcntCloseConnector |
Close a connection to the device |
xcntSendToConnector |
Send an Ethernet frame to the device |
xcntReceiveFromConnector |
Receive an Ethernet frame from the device |
xcntSetMediaStatus |
Change the media status of the Connector Device |
xcntOpenConnector
Establish a connection to the Connector Device
#include <xcnt_api.h>
typedef void* XCNTHANDLE;
int xcntOpenConnector(
const char *cntName,
const BYTE *macAddr,
DWORD phyParams,
DWORD reserved,
DWORD timeoutMs,
XCNTHANDLE *pHandle
);
Parameters:
cntName
- The name of the connector device in the INtime Network. For example, the first connector device name will be “xcnt0”.
macAddr
- Specifies the MAC address for the device. Usually, this is the MAC address of the corresponding HPE device.
phyParams
- Specifies the link speed and link duplex mode. If XCNT_SPEED_NONE is specified, then the link status of the XCNT device is set to “down”, and XCNT will not forward packets sent from istack (xcntReceiveFromConnector will not get new packets). Once the device is open, then xcntSetMediaStatus can be used to change the media status later. The phyParams parameter (other than XCNT_SPEED_NONE) doesn’t affect the behavior of the connector device, but it changes the appearance of the device, i.e. the ifconfig command will display the speed and duplex mode specified by phyParams for the connector device.
reserved
- Must be 0.
timeoutMs
- Timeout value in milliseconds.
pHandle
- A pointer to a handle.
Return Values
E_OK
- success
E_PARAM
- parameter error
E_MEM
- out of physical memory
E_VMEM
- out of virtual memory
E_TIME
- timed out waiting for connector
xcntCloseConnector
Close a connection.
#include <xcnt_api.h>
int xcntCloseConnector( XCNTHANDLE *pHandle );
Parameters:
pHandle
- A pointer to a handle previously returned from a call to xcntOpenConnector.
Return Values
E_OK
- success
E_PARAM
- parameter error
xcntSendToConnector
Send an Ethernet frame to the device.
#include <xcnt_api.h>
int xcntSendToConnector(
XCNTHANDLE handle,
const BYTE *pBuffer,
DWORD bufferLength
);
Parameters:
handle
- A handle previously returned from a call to xcntOpenConnector.
pBuffer
- A pointer to an Ethernet frame data buffer.
bufferLength
- Length of the frame including the Ethernet header (destination address, source address and ether-type) but excluding FCS (checksum).
Return Values
E_OK
- success
E_PARAM
- parameter error
E_STATE
- out of synch with the stack
EH_GENERAL_PROTECTION
- a fault occurred
xcntReceiveFromConnector
Receive an Ethernet frame from the device.
#include <xcnt_api.h>
int xcntReceiveFromConnector(
XCNTHANDLE handle,
BYTE *pBuffer,
DWORD *pBufferLength,
DWORD timeoutMs
);
Parameters:
handle
- A handle previously returned from a call to xcntOpenConnector.
pBuffer
- A pointer to an Ethernet frame data buffer. The buffer must be at least 1514 bytes long.
pBufferLength
- A pointer to a variable where the length of the returned frame will be stored. The length includes the Ethernet header (destination address, source address and ether-type) but excludes the FCS (checksum).
timeoutMs
- A timeout value in milliseconds. The caller will be blocked until it receives a frame or the timeout expires.
Return Values
E_OK
- success
E_PARAM
- parameter error
E_TIME
- timed out waiting for data
E_CANCELED
- device closed
E_EMPTY_ENTRY
- packet expected but none in ring buffer
EH_GENERAL_PROTECTION
- a fault occurred
xcntSetMediaStatus
Change the Media Status for the connector device.
#include <xcnt_api.h>
int xcntSetMediaStatus(
XCNTHANDLE handle,
DWORD phyParams
);
Parameters:
handle
- A handle previously returned from a call to xcntOpenConnector.
phyParams
- Specifies the link speed and link duplex mode. If XCNT_SPEED_NONE is specified, then the list status is changed to “down”.
Return Values
E_OK
- success
E_PARAM
- parameter error
Requirements
Versions |
Link to |
INtime 4.2 |
xcntif.lib |